Have you ever tried to scrape or automate interactions with a website, only to be stymied by Cloudflare bot protection? Those impenetrable CAPTCHAs and browser checks can bring your web scraping efforts to a halt.
But what if you could bypass Cloudflare altogether? In this article, we'll explore how to use Python and libraries like undetected-chromedriver to stealthily scrape sites protected by Cloudflare.
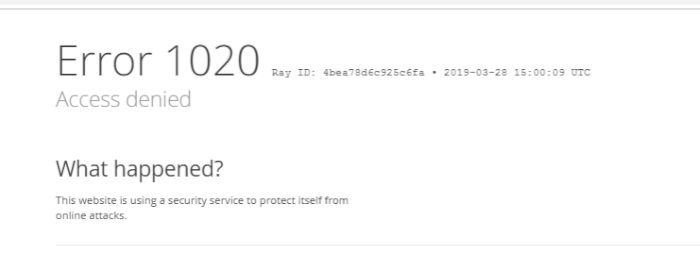
Overview of Cloudflare Bot Protection
Cloudflare is a content delivery network and DDoS protection service used by millions of websites. It also provides bot detection and mitigation capabilities, presenting challenges for scrapers.
When you try to interact with a Cloudflare-enabled site, it can detect bots through JavaScript challenges and browser fingerprinting. If it determines you are a bot, you may face infinite CAPTCHAs or find yourself blocked entirely.
Stealthily Bypassing Cloudflare with undetected-chromedriver
To bypass Cloudflare's protections, we need to fool it into thinking our scraper is a real human visitor. Here's where the Python library undetected-chromedriver comes in handy.
undetected-chromedriver is a Selenium-based Chrome driver that can mimic real human browser behaviors and evade bot mitigation services.
from undetected_chromedriver import Chrome
chrome = Chrome()
chrome.get("<https://example.com>")
By using undetected-chromedriver instead of regular chromedriver, our script can stealthily navigate Cloudflare-protected sites without raising any red flags.
Some key advantages of undetected-chromedriver include:
Putting It All Together to Bypass Cloudflare
Let's walk through an example script leveraging undetected-chromedriver to scrape a Cloudflare-protected site:
from undetected_chromedriver import Chrome
import time
chrome = Chrome()
# Navigate to target url
chrome.get("<https://example.com>")
# Wait for some time to avoid bot detection
time.sleep(10)
# Extract data from site using Selenium
data = chrome.find_element_by_id("data")
print(data.text)
chrome.close()
The key steps are:
- Import undetected-chromedriver and create a Chrome instance
- Navigate to the target URL
- Wait briefly to appear human
- Use Selenium to extract data from the site
- Close the browser
Because we are using undetected-chromedriver instead of regular chromedriver, Cloudflare sees us as a real visitor and does not block our scraping efforts.
Conclusion
By leveraging tools like undetected-chromedriver, we can scrape and automate websites protected by Cloudflare's bot mitigation systems. The techniques covered in this article should give you a template for stealthy and stable web scraping, even on heavily fortified sites.
Rather than building and managing your own cloudfare bypassing infrastructure, services like Proxies API handle all of this complexity for you.
With Proxies API, you make a simple API request with the target URL. It will handle:
And return the rendered HTML. No need to orchestrate the numerous steps required for reliable captcha solving.
For example:
curl "http://api.proxiesapi.com/?key=API_KEY&render=true&url=https://targetpage.com"
This takes care of all the headaches of automation. No proxies, browsers, or captcha solving services to manage.
Proxies API offers 1000 free API calls to get started. Check it out if you need to integrate robust captcha solving and proxy rotation in your projects.