I'll go through step-by-step how to scrape and extract data from eBay listings using Rust. I'll be sure to explain each part of the code.
Setup
We need two crates:
reqwest = { version = "0.11", features = ["blocking"] }
select = "0.5"
And import them:
use reqwest;
use select::document::Document;
Define the URL and a browser User-Agent:
let url = "<https://www.ebay.com/sch/i.html?_nkw=baseball>";
let user_agent = "Mozilla/5.0 ..."; // Use your browser's UA
Fetch the HTML
Make a blocking GET request:
let resp = reqwest::blocking::get(url)
.header("User-Agent", user_agent)
.send()?
.text()?;
Pass the user-agent header and handle errors with
Parse the HTML
let doc = Document::from(resp.as_str());
Load into a
Extract Data
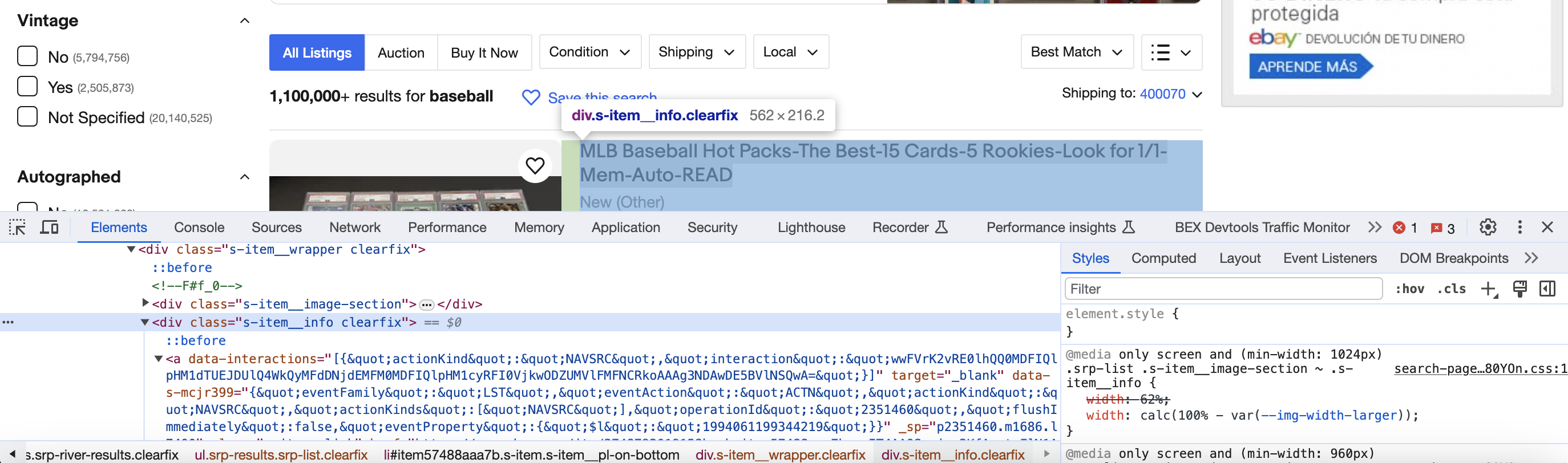
Loop through listings:
for listing in doc.find(Class("s-item__info")) {
let title = listing.find(Class("s-item__title")).text();
let url = listing.find(Class("s-item__link")).attr("href");
let price = listing.find(Class("s-item__price")).text();
// Extract all fields
}
Use
Print Results
println!("Title: {}", title);
println!("URL: {}", url);
println!("Price: {}", price);
println!("{:=^50}", ""); // Separator
Full Code
use reqwest;
use select::document::Document;
let url = "https://www.ebay.com/sch/i.html?_nkw=baseball";
let user_agent = "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/98.0.4758.102 Safari/537.36";
let resp = reqwest::blocking::get(url)
.header("User-Agent", user_agent)
.send()?
.text()?;
let doc = Document::from(resp.as_str());
for listing in doc.find(Class("s-item__info")) {
let title = listing.find(Class("s-item__title")).text();
let url = listing.find(Class("s-item__link")).attr("href");
let price = listing.find(Class("s-item__price")).text();
let details = listing.find(Class("s-item__subtitle")).text();
let seller = listing.find(Class("s-item__seller-info-text")).text();
let shipping = listing.find(Class("s-item__shipping")).text();
let location = listing.find(Class("s-item__location")).text();
let sold = listing.find(Class("s-item__quantity-sold")).text();
// Print all fields
println!("Title: {}", title);
println!("URL: {}", url);
println!("Price: {}", price);
println!("Details: {}", details);
println!("Seller: {}", seller);
println!("Shipping: {}", shipping);
println!("Location: {}", location);
println!("Sold: {}", sold);
println!("{:=^50}", "");
}