Web scraping is the process of extracting data from websites through an automated procedure. It allows you to harvest vast amounts of web data that would be infeasible to gather manually.
Python developers frequently use a library called Beautiful Soup for web scraping purposes. Beautiful Soup transforms complex HTML and XML documents into Pythonic data structures that are easy to parse and navigate.
In this comprehensive tutorial, you'll learn how to use Beautiful Soup to extract data from web pages.
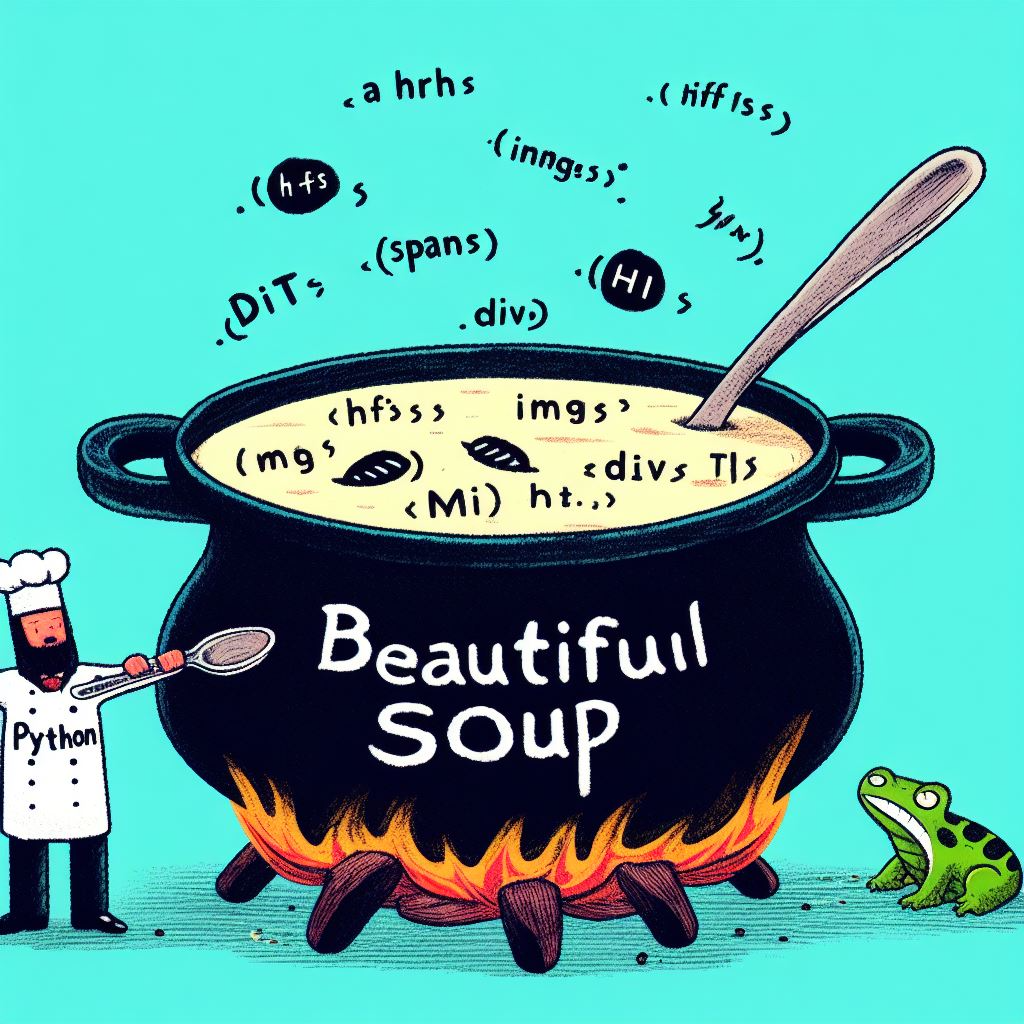
Overview of Web Scraping
Before diving into Beautiful Soup specifics, let's review some web scraping basics.
Web scrapers automate the process of pulling data from sites. They enable you to gather information at scale, saving an enormous amount of manual effort. Common use cases for scrapers include:
Web scraping can be done directly in the browser using developer tools. However, serious scraping requires an automated approach.
When scraping, it's important to respect site terms of use and avoid causing undue load. Make sure to throttle your requests rather than slamming servers.
Now let's look at how Beautiful Soup fits into the web scraping landscape.
Introduction to Beautiful Soup
Beautiful Soup is a Python library designed specifically for web scraping purposes. It provides a host of parsing and navigation tools that make it easy to loop through HTML and XML documents, extract the data you need, and move on.
Key features of Beautiful Soup include:
You can install Beautiful Soup via pip:
pip install beautifulsoup4
The library has dependencies on lxml and html5lib, which will be installed automatically.
With Beautiful Soup installed, let's walk through hands-on examples of how to use it for web scraping.
Creating the Soup Object
To use Beautiful Soup, you first need to import it and create a "soup" object by parsing some HTML or XML content:
from bs4 import BeautifulSoup
html_doc = """
<html>
<body>
<h1>Hello World</h1>
</body>
</html>
"""
soup = BeautifulSoup(html_doc, 'html.parser')
The soup object encapsulates the parsed document and provides methods for exploring and modifying the parse tree.
You can parse HTML/XML from files, URLs, or already-fetched page content like we did above.
Understanding the HTML Tree
Before diving into BeautifulSoup, it's helpful to understand how HTML pages are structured as a tree.
HTML documents contain nested tags that form a hierarchical tree-like structure. Here is a simple example structure:
<html>
<head>
<title>Page Title</title>
</head>
<body>
<h1>Heading</h1>
<p>Paragraph text</p>
</body>
</html>
This page has a root
You can visualize this document as a tree:
html
/ \\
head body
/ | \\
title h1 p
The tree-like structure of HTML allows elements to have parent-child relationships. For example:
When parsing HTML with BeautifulSoup, you can leverage these hierarchical relationships to navigate up and down the tree to extract data. Methods like
Understanding this tree structure helps when conceptualizing how to search and traverse HTML pages with BeautifulSoup.
Searching the Parse Tree
Once you've created the soup, you can search within it using a variety of methods. These allow you to extract precisely the elements you want.
Finding Elements by Tag Name
To find tags by name, use the
h1_tag = soup.find('h1')
all_p_tags = soup.find_all('p')
This finds the first or all instances of the given tag name.
Finding Elements by Attribute
You can also search for tags that contain specific attributes:
soup.find_all('a', class_='internal-link')
soup.find('input', id='signup-button')
Attributes can be string matches, regular expressions, functions, or lists.
CSS Selectors
Beautiful Soup supports CSS selectors for parsing out page elements:
# Get all inputs
inputs = soup.select('input')
# Get first H1
h1 = soup.select_one('h1')
These selectors query elements just like in the browser.
Searching by Text Content
To find elements containing certain text, use a string or regular expression:
soup.find_all(text='Hello')
soup.find_all(text=re.compile('Introduction'))
This locates text matches irrespective of HTML tags.
Search Filters
Methods like
def is_link_to_pdf(tag):
return tag.name == 'a' and tag.has_attr('href') and tag['href'].endswith('pdf')
soup.find_all(is_link_to_pdf)
Filters give you complete control over complex search logic.
Parsing XML Documents
Beautiful Soup can also parse XML documents. The usage is similar, just specify "xml" instead of "html.parser" when creating the soup:
xml_doc = """
<document>
<title>Example XML</title>
<content>This is example XML content</content>
</document>
"""
soup = BeautifulSoup(xml_doc, 'xml')
You can then search and navigate the XML tree using the same methods.
Navigating the Parse Tree
Beautiful Soup provides several navigation methods to move through a document once you've zeroed in on elements.
Parents and Children
Move up to parent elements using
link = soup.find('a')
parent = link.parent
And down to children with
parent = soup.find(id='main-section')
parent.contents # direct children
parent.children # generator of children
Siblings
Access sibling elements alongside each other using
headline = soup.find(class_='headline')
headline.next_sibling # next section after headline
headline.previous_sibling # section before headline
Siblings are powerful for sequentially processing elements.
Traversing the HTML Tree
Going Down: Children
You can access child elements using the
body = soup.find('body')
for child in body.contents:
print(child)
for child in body.children:
print(child)
This allows you to iterate through direct children of an element.
Going Up: Parents
To access parent elements, use the
title = soup.find('title')
print(title.parent)
# <head>...</head>
You can call
Sideways: Siblings
Sibling elements are at the same level in the tree. You can access them using
h1 = soup.find('h1')
print(h1.next_sibling)
# <p>Paragraph text</p>
print(h1.previous_sibling)
# None
You can traverse sideways through siblings to extract related data at the same level.
Using these navigation methods, you can move freely within the HTML document as you extract information.
Extracting Data
Now that you can target elements, it's time to extract information.
Getting Element Text
Use the
paragraphs = soup.find_all('p')
for p in paragraphs:
print(p.text)
This strips out all HTML tags and formatting.
Getting Attribute Values
Access tag attributes using square brackets:
links = soup.find_all('a')
for link in links:
url = link['href'] # get href attribute
text = link.text
print(f"{text} -> {url}")
Common attributes to extract include
Modifying the Parse Tree
Beautiful Soup allows you to directly modify and delete parts of the parsed document.
Editing Tag Attributes
Change attribute values using standard dictionary assignment:
img = soup.find('img')
img['width'] = '500' # set width to 500px
Attributes can be added, modified, or deleted.
Editing Text
Change the text of an element using
h2 = soup.find('h2')
h2.string = 'New headline'
This replaces the entire text contents.
Inserting New Elements
Add tags using
new_tag = soup.new_tag('div')
new_tag.string = 'Hello'
soup.body.append(new_tag)
Deleting Elements
Remove elements with
ad = soup.find(id='adbanner')
ad.decompose() # remove from document
This destroys and removes the matching element from the tree.
Managing Sessions and Cookies
When scraping across multiple pages, you'll need to carry over session state and cookies. Here's how.
Persisting Sessions
Create a session object to persist cookies across requests:
import requests
session = requests.Session()
r1 = session.get('<http://example.com>')
r2 = session.get('<http://example.com/user-page>') # has cookie
Now cookies from
Working with Cookies
You can get, set, and delete cookies explicitly using
# Extract cookies
session.cookies.get_dict()
# Set a cookie
session.cookies.set('username', 'david', domain='.example.com')
# Delete cookie
session.cookies.clear('.example.com', '/user-page')
This gives you full control over request cookies.
Writing Scraped Data
To use scraped data, you'll need to write it to file formats like JSON or CSV for later processing:
Writing to CSV
Use Python's CSV module to write a CSV file:
import csv
with open('data.csv', 'w') as f:
writer = csv.writer(f)
writer.writerow(['Name', 'URL']) # write header
products = scrape_products() # custom scrape function
for p in products:
writer.writerow([p.name, p.url])
Writing to JSON
Serialize scraped data to JSON using
import json
data = scrape_data() # custom scrape function
with open('data.json', 'w') as f:
json.dump(data, f)
This writes clean JSON for loading later.
Handling Encoding
When parsing content from the web, dealing with character encoding is important for extracting clean text.
By default, Beautiful Soup parses documents as UTF-8 encoded. However, pages may use other encodings like ASCII or ISO-8859-1.
You can specify a different encoding when creating the soup:
soup = BeautifulSoup(page.content, 'html.parser', from_encoding='iso-8859-1')
However, Beautiful Soup also contains tools to detect and convert encodings automatically:
Detect Encoding
To detect the encoding of a document, use
from bs4 import UnicodeDammit
dammit = UnicodeDammit(page.content)
print(dammit.original_encoding) # e.g. 'utf-8'
It analyzes the byte patterns at the start of the document.
Convert Encoding
To automatically convert to Unicode, pass the document to
soup = BeautifulSoup(UnicodeDammit(page.content).unicode_markup, 'html.parser')
It will convert from detected encodings like ISO-8859-1 to UTF-8 by default.
With these tools, you can account for varying document encodings when scraping the web and extracting clean text from HTML.
Copying and Comparing Objects
When parsing HTML with Beautiful Soup, you may need to copy soup objects to modify them separately or compare two objects.
Copying
To create a copy of a Beautiful Soup object, use the
original = BeautifulSoup(page)
copy = original.copy()
This creates a detached copy that can be modified independently.
Comparing
To test if two objects contain the same parsed HTML, use the
soup1 = BeautifulSoup(page1)
soup2 = BeautifulSoup(page2)
if soup1 == soup2:
print("Same HTML")
else:
print("Different HTML")
Behind the scenes, the objects are compared by serializing and diffing their HTML.
This can be useful for comparing scraped pages across different times or sources.
Also note that soup objects act like Python dictionaries in many ways, so you can use
if <p> in soup:
print("Contains paragraph tag")
These utilities allow easily working with multiple Beautiful Soup objects when scraping at scale.
Using SoupStrainer
When parsing large HTML documents, you may want to target only specific parts of the page. SoupStrainer allows you to parse only certain sections of a document.
A SoupStrainer works by defining filters that match certain tags and attributes. You can pass it to the BeautifulSoup constructor to selectively parse only certain elements:
from bs4 import SoupStrainer
strainer = SoupStrainer(name='div', id='content')
soup = BeautifulSoup(page, 'html.parser', parse_only=strainer)
This will only parse
You can make the strainer match multiple criteria:
strainer = SoupStrainer(name=['h1', 'p'])
This will parse only
SoupStrainer is useful for scraping large pages where you only need a small section. It avoids parsing and searching through irrelevant parts of the document.
You can pass multiple strainers to parse different sections of a page. Or combine with searching and filtering to further narrow your results.
Error Handling
When writing scraping scripts, you'll encounter errors like missing attributes or tags that should be handled gracefully.
Missing Attributes
To safely access a tag attribute that may be missing, use the
url = link.get('href')
if not url:
# handle missing href
This avoids an AttributeError when the attribute doesn't exist.
Missing Tags
When searching for tags, use exception handling to account for missing elements:
try:
title = soup.find('title').text
except AttributeError as e:
print('Missing title tag')
title = None
This prevents crashes if the expected tag isn't found.
Invalid Markup
You can configure Beautiful Soup to silently ignore bad markup instead of raising exceptions:
soup = BeautifulSoup(page, 'html.parser', recover=True)
It will skip tags that aren't properly formatted or closed.
HTTP Errors
Handle HTTP errors when making requests:
try:
page = requests.get(url)
page.raise_for_status()
except requests.exceptions.HTTPError as e:
print('Request failed:', e)
This catches non-200 status codes.
With proper error handling, your scrapers will be more robust and resilient.
Common Web Scraping Questions
Here are answers to some common questions about web scraping using Beautiful Soup:
How can I extract data from a website using Python and BeautifulSoup?
Use the
What are some good web scraping tutorials for beginners?
Some good beginner web scraping tutorials using Python cover inspecting the page DOM, installing libraries like
How do I handle dynamic websites with Javascript?
Beautiful Soup itself only parses static HTML. For dynamic pages, you'll need a browser automation tool like Selenium to load the Javascript and render the full page before passing it to BeautifulSoup.
What are some common web scraping mistakes?
Some mistakes to avoid are hammering servers with too many requests, failing to check for robots.txt restrictions, not throttling requests, scraping data you don't have rights to use, and not caching pages that change infrequently.
How can I scrape data from pages that require login?
Use the
How do I bypass captchas and blocks when scraping?
Options include rotating user agents and proxies to mask scrapers, solving captchas manually or with services, respecting crawl delays, and using headless browsers like Selenium to mimic human behavior.
This is a lot to learn and remember. Is there a cheat sheet for this?
Glad you asked. We have created a really exhaustive cheat sheet for beautiful soup here.
Conclusion
Beautiful Soup is a handy library for basic web scraping tasks in Python. It simplifies parsing and element selection, enabling you to get up and running quickly.
However, Beautiful Soup has some limitations:
For more heavy-duty web scraping projects, you will likely need additional tools and services beyond Beautiful Soup itself:
This is where a service like Proxies API can help take your web scraping efforts to the next level.
With Proxies API, you get all the necessary components for robust web scraping in one simple API:
The Proxies API takes care of all the proxy rotation, browser automation, captcha solving, and other complexities behind the scenes. You can focus on writing your Beautiful Soup parsing logic to extract data from the rendered pages it delivers.
If you are looking to take your web scraping to the next level, combining the simplicity of BeautifulSoup with the power of Proxies API is a great option to consider.